You may have already known how important it is to set a solid and secure password for each website. There is a bright future for authentication at least till we have passkeys. Until then we have to keep using passwords and maybe use a password manager to keep all those passwords safe.
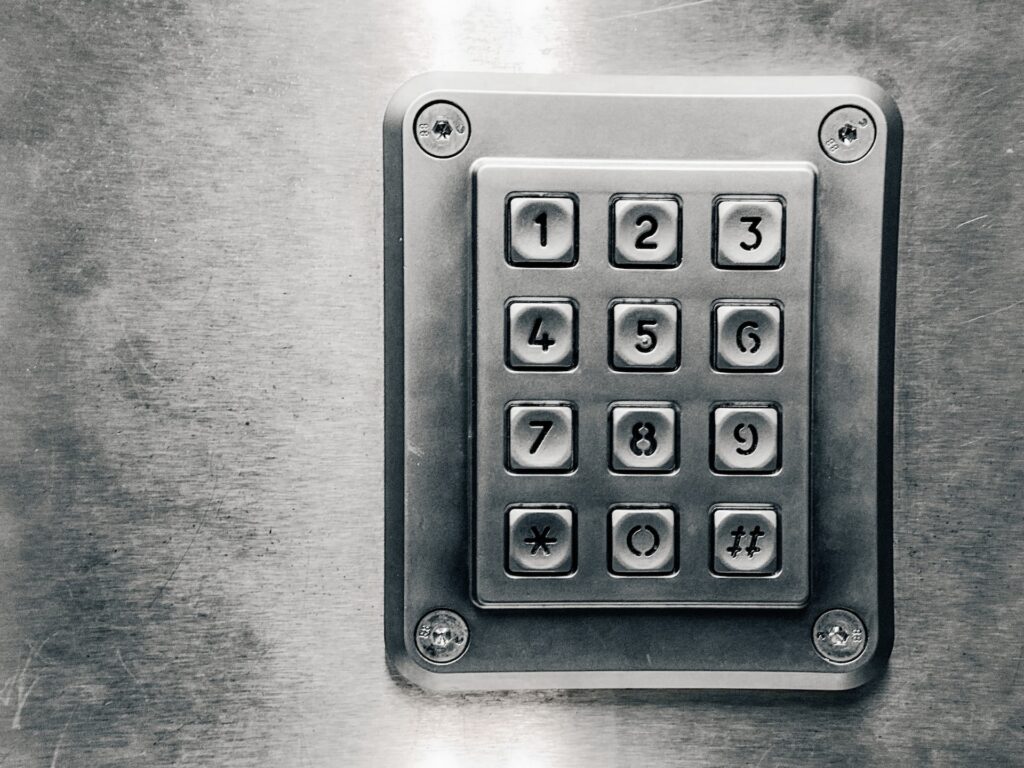
Everyone in their life must have come to a situation where a website keeps on asking for a secure password and you can’t generate it. So here is the simple Python password generator
It will generate a random password, based on the characters you have specified. You can copy the python code below and keep on generating passwords.
How to use it ?
You just need to copy the script and paste it into a file on your server or windows machine. If you are using it as server then you can save this script as passwordgenerator.py
then simply run the command below.
$ python3 passwordgenerator.py
You should get the output below.
Welcome to the PyPassword Generator! How many characters would you like in your password? 25 Your random password is: 159&2!I)Bg&%+u#2Zu1J&i7v7
So, just hover your mouse on the code and it will show you the button to copy code 🙂
# Password Generator Project import random # setting up the characters, numbers and symbols to choose from. letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z'] numbers = ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9'] symbols = ['!', '#', '$', '%', '&', '(', ')', '*', '+'] # Getting the input from user print("Welcome to the PyPassword Generator!") # Here we will ask user how much character he wants in his password. characters = int(input("How many characters would you like in your password?\n")) # Making sure that characters are between 1 and 50, else a user can enter a very large number and break down a server. if characters > 50 or characters < 1: print("You need to choose number between 1 and 50") exit() # Since we are choosing 3 different characters in password, I making sure that number is divisible 3. # Hence, I will get the remainder and subtract it from the main number. remainder = characters % 3 divisible_number = characters - remainder # I am making sure that each character have similar numbers. nr_letters = round((divisible_number / 3) + remainder) nr_symbols = round(divisible_number / 3) nr_numbers = round(divisible_number / 3) # creating a list of characters using for loop. password_list = [] for number in range(1, nr_letters + 1): password_list.append(random.choice(letters)) for number in range(1, nr_symbols + 1): password_list.append(random.choice(symbols)) for number in range(1, nr_numbers + 1): password_list.append(random.choice(numbers)) # at this point if you just print password_list will just print list # below code will help in randomizing the password in the correct format in the list.. random.shuffle(password_list) # setting the password as blank initially. password = "" # Coverting list into String for char in password_list: password += char # Printing Final Password print(f" Your random password is: {password}")
Hopefully, you have liked the Python password generator. Let me know if you have any suggestions or feedback.